📢 어렵고 정석적인 개념 설명보다는 저같은 초보자도 이해하기 쉽게 정리하는 것을 원칙으로 포스팅하고 있습니다. 😄
[SCSS] @for를 이용해서 반복되는 스타일 쉽게 적용하기
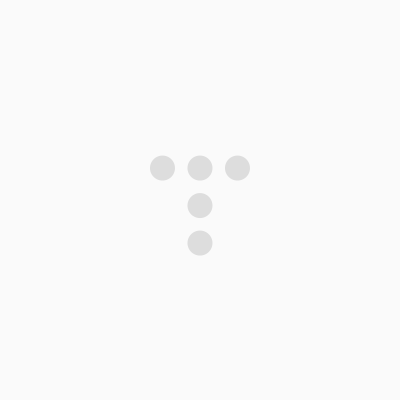
Sass(SCSS)
2022. 9. 19. 11:22
문제
우리가 웹의 마크업 작업들을 하다 보면, 반복되는 스타일의 요소 배열들이 이따금씩 나타나는데 이때마다 우리는 nth-child 혹은 nth-of-type 등과 같은 가상선택자를 이용해서 하나씩 따로따로 스타일을 줬어야만 했다.
그 요소들이 2~3개라면 괜찮은데 5개 이상의 요소라면 코드를 치는 사람도, 보는 사람도 지저분하게 보일 수 있다. 이 때, 자바스크립트의 for문처럼 반복되는 스타일을 하나로 묶어버릴 수 있는 기능이 바로 SCSS의 @for문이다.
방법
종료 직전까지 반복 (from A to B)
/* SCSS */
@for $i from 1 to 6 {
div:nth-child(#{$i}){
width: $i * 100px;
background-image: url("./images/icon_#{$i}");
padding: 0 #{$i}px;
}
}
/* CSS */
div:nth-child(1) {
width: 100px;
background-image: url("./images/icon_1");
padding: 0 1px;
}
div:nth-child(2) {
width: 200px;
background-image: url("./images/icon_2");
padding: 0 2px;
}
div:nth-child(3) {
width: 300px;
background-image: url("./images/icon_3");
padding: 0 3px;
}
div:nth-child(4) {
width: 400px;
background-image: url("./images/icon_4");
padding: 0 4px;
}
div:nth-child(5) {
width: 500px;
background-image: url("./images/icon_5");
padding: 0 5px;
}
첫 번째 방식은 @for 변수 from 시작번호 to 종료번호로, 종료번호 직전까지 반복시키는 방식이다.
변수를 사용할 때는 항상 $를 앞에 붙여주고, 단독으로 사용할 때는 그냥 $i(변수명 i)로 써도 되지만 nth-child와 같은 가상선택자, url 주소, px과 함께 사용 등 무언가와 함께 사용될 때는 #{$i}로 써야 한다.
종료까지 반복 (from A through B)
/* SCSS */
@for $i from 1 through 6 {
div:nth-child(#{$i}){
width: $i * 100px;
background-image: url("./images/icon_#{$i}");
padding: 0 #{$i}px;
}
}
/* CSS */
div:nth-child(1) {
width: 100px;
background-image: url("./images/icon_1");
padding: 0 1px;
}
div:nth-child(2) {
width: 200px;
background-image: url("./images/icon_2");
padding: 0 2px;
}
div:nth-child(3) {
width: 300px;
background-image: url("./images/icon_3");
padding: 0 3px;
}
div:nth-child(4) {
width: 400px;
background-image: url("./images/icon_4");
padding: 0 4px;
}
div:nth-child(5) {
width: 500px;
background-image: url("./images/icon_5");
padding: 0 5px;
}
div:nth-child(6) {
width: 600px;
background-image: url("./images/icon_6");
padding: 0 6px;
}
두 번째 방식은 @for 변수 from 시작번호 through 종료번호로, 종료번호까지(포함) 반복시키는 방식이다.
쉽게 말하면 to는 미만, through는 이하라고 생각하면 된다.
1. @for 변수 from 시작번호 to 종료번호 : 종료번호 직전까지
2. @for 변수 from 시작번호 through 종료번호 : 종료번호 포함해서
3. 변수를 단독으로 사용할 땐 $변수만 쓰고, 다른 문자와 함께 사용할 땐 #{$변수}으로 사용
'Sass(SCSS)' 카테고리의 다른 글
[SCSS] @content로 @mixin에 스타일 속성 추가하기 (0) | 2022.08.19 |
---|---|
[SCSS] 색상 투명도를 조절하는 컬러 함수 transparentize() (0) | 2022.08.16 |
[SCSS] 다중 변수 선언 get-map() 함수 사용하기 (0) | 2022.06.13 |
[SCSS] @extend로 연관성있는 선택자 속성 가져오기 (0) | 2022.06.13 |
[SCSS] @mixin과 @include로 스타일 그룹 사용하기 (0) | 2022.06.07 |