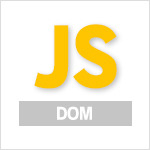
DOM 추가/삭제하기 // 1. div라는 태그를 만든다. const wrapDiv = document.createElement('div'); // 2. 만든 div에 text를 넣는다. (2가지 방법) wrapDiv.textContent = 'Hello World!'; wrapDiv.append('Hello World!'); // 3. 만든 div의 자식 요소로 넣을 h1 태그를 만든다. const txtH1 = document.createElement('H1'); // 4. 만든 h1에도 text를 넣어준다. txtH1.textContent = '안녕하세요.'; // 5. 만든 h1을 div의 자식 요소로 추가해준다. (2가지 방법) wrapDiv.append(txtH1); wrapDiv.append..
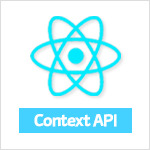
What App.js - List.js - Item.js의 순서대로 자식 컴포넌트가 있다고 가정해보자. 즉, App.js가 최상위 부모 컴포넌트고 그 밑으로는 List.js, 또 그 밑으로는 Item.js 컴포넌트가 있는 것이다. 만약, App 컴포넌트의 state를 Item 컴포넌트에게 전달하려면 어떻게 해야할까? 기존의 일반적인 방법으로는 App이 List 컴포넌트에게 props를 먼저 전달하고, 전달받은 props를 다시 Item 컴포넌트로 전달하는 과정을 거쳐야 했다. List 컴포넌트는 해당 props를 쓰지도 않는데 그저 Item 컴포넌트의 부모 컴포넌트라는 이유로 props를 전달하는 다리 역할만 한 것이다. 이것은 매우 비효율적이다. 지금은 3개의 컴포넌트지만, 10개라면? 생각만 해도 이..
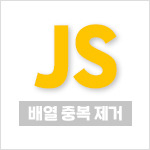
What 특정 배열안의 원소가 [1, 2, 3, 1, 2, 3]이 있다고 하자. 그런데, 나는 1, 2, 3이 반복이 되므로 중복된 원소는 제거해서 [1, 2, 3]이라는 깔끔한 배열로 만들고 싶다. 그럴 땐, filter()와 indexOf()을 활용하여 쉽게 중복 원소를 제거할 수 있다. How const arr = [3, 2, 5, 3, 2, 6, 5, 4]; const filterArr = arr.filter((el, idx) => { return arr.indexOf(el) === idx; }) console.log(filterArr); // [3, 2, 5, 6, 4] 지저분한 중복 원소들로 가득찬 arr 배열이 있다. 이제 다음 단계들을 거쳐서 깔끔하게 중복 원소들을 제거해보자. 1) 우선 ..
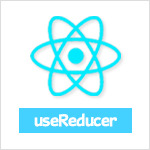
What useReducer()는 useState() 대신 사용하는 Hook 함수이며, 다수의 상태를 관리하는 데 유용한 함수이다. useReducer() 함수를 실행하기 전, 먼저 reducer 함수를 만들어줘야 한다. reducer는 상태 객체와 행동 객체를 인자로 받아서 새로운 상태 객체를 반환하는 함수이다. How const Test = ({ num = 5 }) => { const reducer = (state, action) => { switch (action.type){ case 'increase': return state + action.num; case 'decrease': return state - action.num; case default: return state; } } const ..
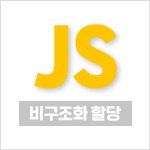
배열의 비구조화 할당 배열 선언 및 호출 const [apple, banana, grape] = ['apple1', 'banana1', 'grape1']; console.log(apple); // apple1 console.log(banana); // banana1 console.log(grape); // grape1 변수명과 값을 배열 형식으로 한꺼번에 선언할 수 있다. 나머지 패턴 const fruitList = ['apple', 'banana', 'grape']; const [apple1, ...rest] = fruitList; console.log(apple1); // apple console.log(rest); // ['banana', 'grape'] 전개 연산자를 통해 나머지 변수들을 따로 분..